Join Our Giveaway!
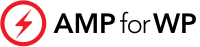
WordPress is renowned for its easy-to-use interface and a plethora of features that make it an ideal content management system. It has also evolved to offer much more than a blogging platform, and today it powers a large chunk of the internet’s websites. Part of this growth can be attributed to the extendability of WordPress through the use of themes and plugins.
The WPDB class is a set of PHP classes provided by WordPress to interact with and manipulate the database. It’s an essential tool for developers who wish to create, read, update, or delete data from the WordPress database in their plugins or themes.
This blog post is a comprehensive guide to using the WPDB class in PHP.
The WPDB class is automatically included in the WordPress core, which means there’s no need to include it manually in your script. You can use the global $wpdb object, which is an instance of the WPDB class.
phpCopy codeglobal $wpdb;
With this object, you can now access all the methods and variables that the WPDB class provides.
CRUD stands for Create, Read, Update, and Delete – these are the fundamental operations that you can perform on a database.
To insert data into a table, you can use the insert()
method. Here’s how you would insert data into a custom table:
phpCopy code$wpdb->insert('table_name', array(
'column1' => 'value1',
'column2' => 'value2'
));
To fetch data from a table, you can use several methods. The get_results()
method is probably the most common one. Here is how you would get all rows from a custom table:
phpCopy code$results = $wpdb->get_results("SELECT * FROM table_name");
Updating data in a table is also straightforward. You can use the update()
method. Here’s an example:
phpCopy code$wpdb->update('table_name', array(
'column1' => 'new_value1' // String or integer
), array(
'ID' => 1
));
Deleting data from a table can be achieved using the delete()
method. Here is how you would delete a row from a custom table:
phpCopy code$wpdb->delete('table_name', array(
'ID' => 1
));
It’s crucial to always secure your queries to prevent SQL injection attacks. The WPDB class provides several ways to achieve this.
The simplest method is using placeholders in your SQL queries. You use the %s
placeholder for strings, %d
for integers, and %f
for floats.
phpCopy code$sql = $wpdb->prepare("SELECT * FROM table_name WHERE id = %d", $id);
$results = $wpdb->get_results($sql);
In this example, the %d
placeholder is replaced with the value of $id
and it will be treated as an integer.
Lastly, if you need to debug your queries, WordPress provides a method for that as well. You can use $wpdb->last_query
to see the last query that was sent to the database, $wpdb->last_error
to see the last error that occurred, and $wpdb->show_errors()
to display all SQL errors.
WordPress’s WPDB class also supports meta queries, which are incredibly handy when dealing with complex search filters.
Here’s an example of using a meta query:
phpCopy code$args = array(
'meta_query' => array(
array(
'key' => 'color',
'value' => 'blue',
'compare' => '='
)
)
);
$query = new WP_Query($args);
In this example, we are querying all posts where the ‘color’ metadata is ‘blue’.
While the WPDB class offers a wide range of methods for common operations, there may be occasions when you need to write a custom SQL query. The query()
method allows you to execute any SQL query.
phpCopy code$wpdb->query("
DELETE FROM table_name
WHERE column1 = 'value'
");
The query()
method is a lower-level method and does not provide the level of abstraction or security that other methods do. Therefore, it should be used sparingly and carefully.
When dealing with large amounts of data, it’s often helpful to paginate the results. You can use the get_var()
method to fetch the total number of rows and then calculate the number of pages.
phpCopy code$total_rows = $wpdb->get_var("SELECT COUNT(*) FROM table_name");
$items_per_page = 20;
$total_pages = ceil($total_rows / $items_per_page);
WordPress provides a caching mechanism that can help improve the performance of your database queries. This is especially helpful when you have expensive queries that don’t change very often. WordPress uses a technique called “object caching” to store database query results which you can utilize by calling wp_cache_set()
and wp_cache_get()
.
phpCopy code$cache_key = 'my_custom_query';
$results = wp_cache_get($cache_key);
if ($results === false) {
$results = $wpdb->get_results("SELECT * FROM table_name");
wp_cache_set($cache_key, $results);
}
In this example, the results of the query are cached under the key ‘my_custom_query’. The next time this code is run, it will first try to get the results from the cache. If the results are not in the cache, it will run the query and then store the results in the cache.
We’ve explored the advanced features of the WPDB class, including writing custom queries, handling meta queries, paginating results, and leveraging WordPress’s caching mechanisms to speed up our queries.
The WPDB class is a powerful tool in any WordPress developer’s toolkit. With a bit of practice, you’ll be able to create more efficient and safer database interactions for your WordPress themes and plugins. As always, ensure your queries are safe and your data is secure.
Remember, powerful tools require responsible use. Use the WPDB class wisely, always double-check your SQL, and your WordPress site will continue to run smoothly and securely. Happy coding!